"Autonomous low-ops Kubernetes for clusters, workstations, edge and IoT"
Microk8s is a simple way to launch single node kubernetes environment for local development and/or testing and learning purposes for devops. It is a fast, small, cheap k8s for CI/CD.
Minikube is a similair tool to get a kubernetes up and running locally, but with one big difference, MiniKube spins up a VM and runs it in the VM. Microk8s doesn't need a VM, which means you get a lot more resources at your disposal. VM's are pretty heavy on a laptop.
So it sounds good to me :D Let's play with it on my smartos server.
1: Creating a KVM Ubuntu instance
First you need a KVM running with Ubuntu, I used the following setup, create a file k8s-micro.json
:
{
"brand": "bhyve",
"alias": "bionic-k8-master",
"ram": "2048",
"vcpus": "2",
"resolvers": [
"8.8.8.8"
],
"nics": [
{
"nic_tag": "admin",
"gateway": "192.168.1.1",
"netmask": "255.255.255.0",
"ip": "192.168.1.100",
"model": "virtio",
"primary": true
}
],
"disks": [
{
"image_uuid": "c9db249c-93ba-4507-9fa4-b4d0f81265fc",
"boot": true,
"model": "virtio"
}
],
"customer_metadata": {
"root_authorized_keys": "ssh-rsa INSERTKEYHERE somebody@askme",
"cloud-init:user-data": "#cloud-config\n\nresolv_conf:\n nameservers: ['8.8.8.8']\n\nruncmd:\n - curl -s \"https://packages.cloud.google.com/apt/doc/apt-key.gpg\" | apt-key add -\n - echo 'deb http://apt.kubernetes.io/ kubernetes-xenial main' >/etc/apt/sources.list.d/kubernetes.list\n - apt-get update\n - apt-get upgrade -y\n - apt-get install -y docker.io\n - systemctl enable docker\n - systemctl start docker\n - echo 'net.bridge.bridge-nf-call-iptables=1' >>/etc/sysctl.conf\n - sysctl -p\n - swapoff -a\n"
}
}
I have to be honest and explain to you, my default install will automatically install docker with cloud-init.
Lets install it: vmadm install k8s-micro.json
2: Install micro8ks
Login into your new vm and install with snap (current version is 1.18):
# sudo snap install microk8s --classic --channel=1.18/stable
2020-05-19T14:58:03Z INFO Waiting for restart...
microk8s (1.18/stable) v1.18.2 from Canonical✓ installed
Make sure that the user can access the micro8ks without needing to do sudo, my user is ubuntu:
# sudo usermod -a -G microk8s ubuntu
# sudo chown -f -R ubuntu ~/.kube
To make it work, after the commands you need to logout and login again.
3: Checking the status
# microk8s status --wait-ready
microk8s is running
addons:
cilium: disabled
dashboard: disabled
dns: disabled
fluentd: disabled
gpu: disabled
helm: disabled
helm3: disabled
ingress: disabled
istio: disabled
jaeger: disabled
knative: disabled
kubeflow: disabled
linkerd: disabled
metallb: disabled
metrics-server: disabled
prometheus: disabled
rbac: disabled
registry: disabled
storage: disabled
4: Enable the standard services
As a real mimimum I can advise to atleast enable the following plugins:
# microk8s enable dns dashboard registry ingress
5: Check the dashboard
When you have the dashboard enabled you can do the following:
# kubectl proxy --accept-hosts=.* --address=0.0.0.0 &
And open in a browser: http://{{IPOFTHEMACHINE}}:8001/api/v1/namespaces/kube-system/services/https:kubernetes-dashboard:/proxy/
One the first page with the KubeConfig and Token I just pressed the button skip :)
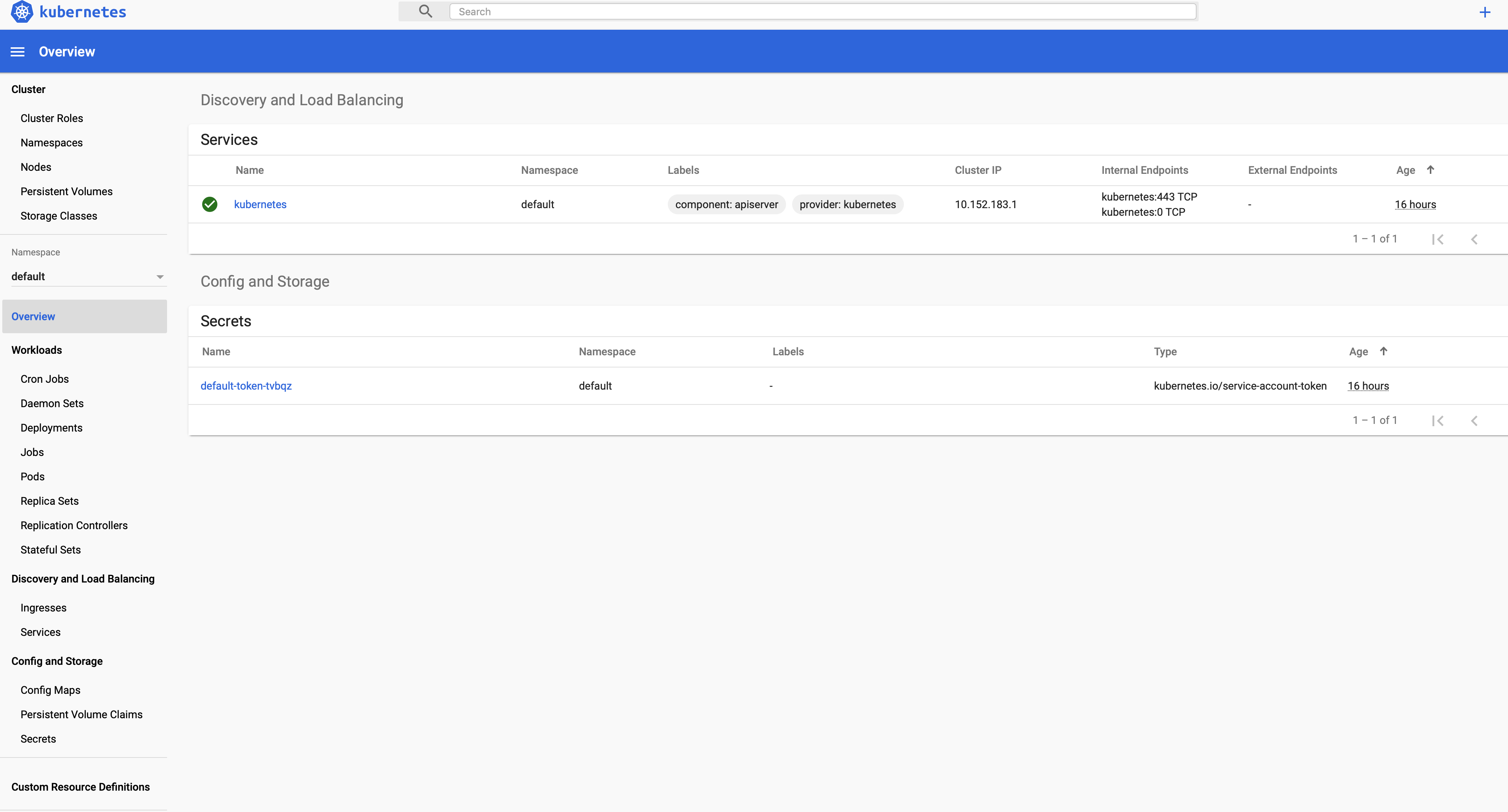
Tips:
You can easily alias kubectl
# sudo snap alias microk8s.kubectl kubectl